250x250
반응형
Notice
Recent Posts
Recent Comments
Link
N
(Leet Code JS)Path Sum II 본문
728x90
반응형
113. Path Sum II
Given the root of a binary tree and an integer targetSum, return all root-to-leaf paths where the sum of the node values in the path equals targetSum. Each path should be returned as a list of the node values, not node references.
A root-to-leaf path is a path starting from the root and ending at any leaf node. A leaf is a node with no children.
Example 1:
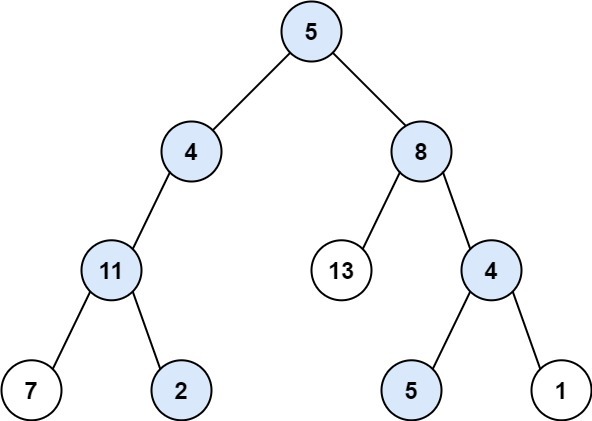
Input: root = [5,4,8,11,null,13,4,7,2,null,null,5,1], targetSum = 22
Output: [[5,4,11,2],[5,8,4,5]]
Explanation: There are two paths whose sum equals targetSum:
5 + 4 + 11 + 2 = 22
5 + 8 + 4 + 5 = 22
Example 2:
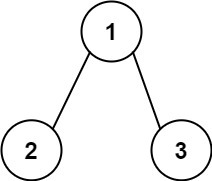
Input: root = [1,2,3], targetSum = 5
Output: []
Example 3:
Input: root = [1,2], targetSum = 0
Output: []
Constraints:
- The number of nodes in the tree is in the range [0, 5000].
- -1000 <= Node.val <= 1000
- -1000 <= targetSum <= 1000
리프 노드이면서 해당 노드까지의 합이 targetSum과 같은 경로를 출력.
dfs를 통해 진행.
dfs 함수
우선 현재까지의 sum이 targetSum과 같은지, 현재 노드의 left와 rigth가 모두 null 인 경우 answer에 현재까지의 경로를 push 후 리턴.
그렇지 않다면 왼쪽과 오른쪽 노드를 dfs 탐색 진행하면 된다.
/**
* Definition for a binary tree node.
* function TreeNode(val, left, right) {
* this.val = (val===undefined ? 0 : val)
* this.left = (left===undefined ? null : left)
* this.right = (right===undefined ? null : right)
* }
*/
/**
* @param {TreeNode} root
* @param {number} targetSum
* @return {number[][]}
*/
const dfs = (node, sumArray, sum, answer, targetSum) => {
if(sum === targetSum && node.left === null && node.right === null){
answer.push(sumArray);
return;
}
if(node.left !== null){
dfs(node.left, [...sumArray, node.left.val], sum + node.left.val, answer, targetSum);
}
if(node.right !== null){
dfs(node.right, [...sumArray, node.right.val], sum + node.right.val, answer, targetSum);
}
};
const pathSum = (root, targetSum) => {
const answer = [];
if(root !== null){
dfs(root, [root.val], root.val, answer, targetSum);
}
return answer;
};
728x90
반응형
'Leet Code 알고리즘' 카테고리의 다른 글
(Leet Code JS)Count and Say (0) | 2021.11.16 |
---|---|
(Leet Code JS)Number of Islands (0) | 2021.11.14 |
(Leet Code JS)Maximum Depth of Binary Tree (0) | 2021.11.14 |
(Leet Code JS)Subdomain Visit Count (0) | 2021.11.06 |
(Leet Code JS)Subarray Sum Equals K (0) | 2021.11.06 |